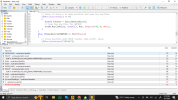
Post automatically merged:
This is the full code
//--- Input parameters
input double LotsPercent = 0.5; // Lot size as a percentage of equity
input int StopLoss = 15; // Stop Loss in pips
input int TakeProfitPips = 0; // Take Profit condition managed by MACD level
input double MACDEntryLevel = -0.0002; // MACD entry level
input double MACDTPLevel = 0.00025; // MACD take profit level\
//--- Global variables
double macdCurrentEURUSD, macdCurrentGBPUSD;
//--- Function to calculate lot size
double CalculateLotSize()
{
double riskPercent = LotsPercent / 100;
double lotSize = AccountFreeMargin() * riskPercent / 1000; // Adjust lot size calculation
return NormalizeDouble(lotSize, 2); // Normalize to 2 decimals
}
//--- Main function: OnTick
void OnTick()
{
// Check timeframes for EURUSD on M1
if(Symbol() == "EURUSD" && Period() == PERIOD_M1)
{
macdCurrentEURUSD = iMACD(NULL, PERIOD_M1, 12, 26, 9, PRICE_CLOSE, MODE_MAIN, 0);
if(macdCurrentEURUSD > MACDEntryLevel)
{
// Check if there's no open position and open buy position
if(PositionsTotal() == 0)
{
double lotSize = CalculateLotSize();
// Open Buy position for EURUSD
trade.Buy(lotSize, Symbol(), Ask, StopLoss*10, 0, NULL);
}
}
else if(macdCurrentEURUSD >= MACDTPLevel)
{
// Close position when MACD reaches take profit level
if(PositionSelect("EURUSD"))
{
trade.PositionClose(Symbol());
}
}
}
// Check timeframes for GBPUSD on M5
if(Symbol() == "GBPUSD" && Period() == PERIOD_M5)
{
macdCurrentGBPUSD = iMACD(NULL, PERIOD_M5, 12, 26, 9, PRICE_CLOSE, MODE_MAIN, 0);
if(macdCurrentGBPUSD > MACDEntryLevel)
{
// Check if there's no open position and open buy position
if(PositionsTotal() == 0)
{
double lotSize = CalculateLotSize();
// Open Buy position for GBPUSD
trade.Buy(lotSize, Symbol(), Ask, StopLoss*10, 0, NULL);
}
}
else if(macdCurrentGBPUSD >= MACDTPLevel)
{
// Close position when MACD reaches take profit level
if(PositionSelect("GBPUSD"))
{
trade.PositionClose(Symbol());
}
}
}
}